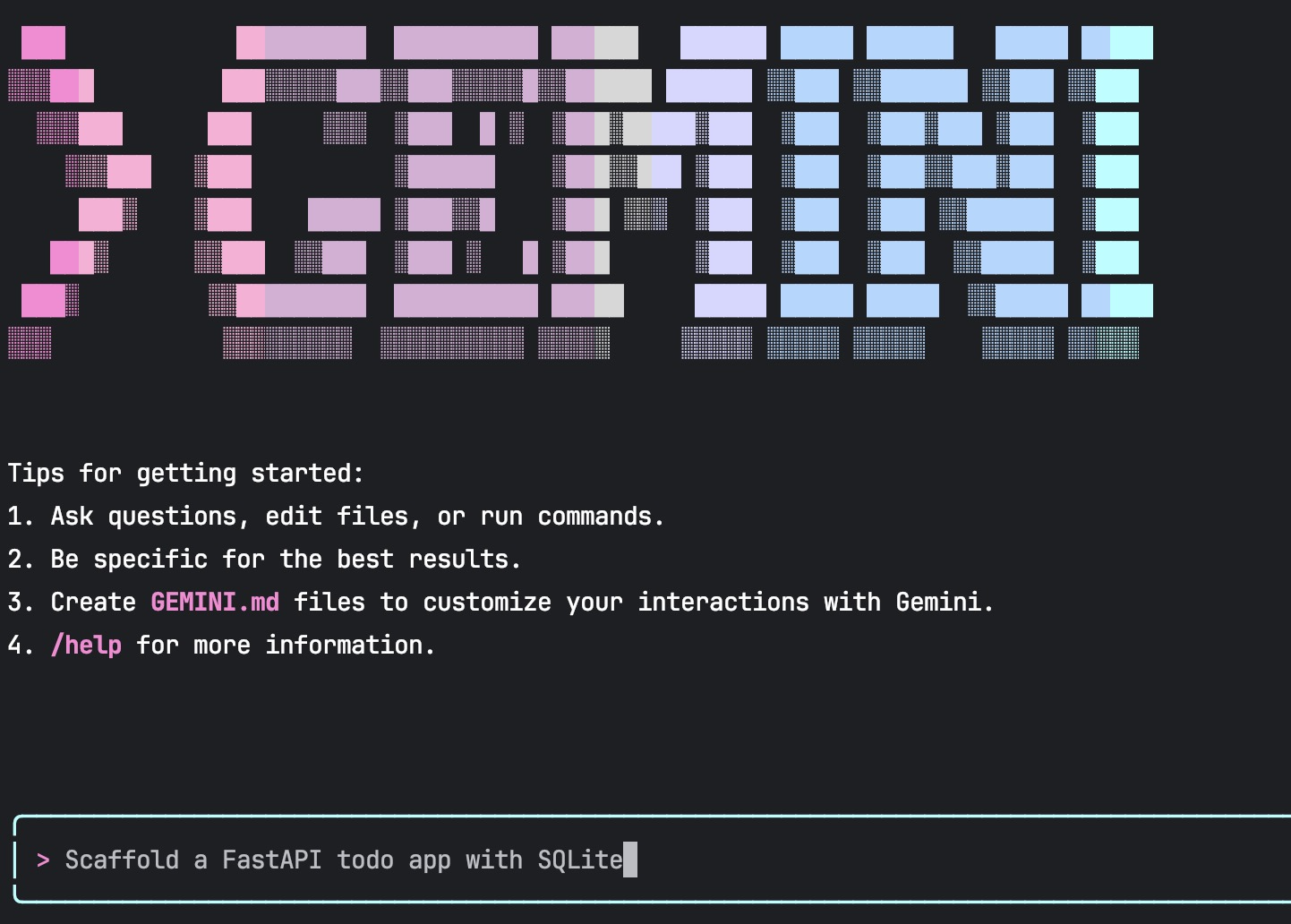
How to Supercharge Your Terminal with Gemini CLI
TL;DR Gemini CLI is Google’s free, open-source AI agent for your terminal. Powered by Gemini 2.5 Pro (with a huge 1 million-token context), it lets you scaffold apps, debug and refactor code, fe...
TL;DR Gemini CLI is Google’s free, open-source AI agent for your terminal. Powered by Gemini 2.5 Pro (with a huge 1 million-token context), it lets you scaffold apps, debug and refactor code, fe...
Sequence-to-sequence (seq2seq) models without attention compress an entire source sentence into a single fixed-length vector, then feed that into a decoder to produce the target sentence. While t...
A modern language model must handle vast contexts, share parameters efficiently, and overcome the vanishing-gradient issues that plagued early RNNs. Count-based n-grams hit a combinatorial wall: s...
If you landed here, you’re probably already using a remote (GPU) for your ML tasks, and you’re sick of git push from your laptop and the pull on your powerful GPU machine. Ask me why I know that, a...
Have you ever tapped on your phone’s keyboard and seen it guess your next word? That “magic” used to (and often still does!) come from n-gram language models—the statistical workhorses that predate...
Modernizing large-scale legacy Java applications in banking is a multifaceted challenge, combining intricate domain logic with stringent compliance and security requirements. Generative AI—especia...
CV-Pilot: A Hands-On Agentic Experiment I’m always on the lookout for ways to push the boundaries of what GenAI can do for productivity. Here’s why I believe agentic AI is the next big leap, wha...
Technical Comparison of Top Agentic AI Frameworks In this article, we skip the basics and get straight to the technical details. We first provide a curated list of popular agentic AI frameworks al...
The landscape of artificial intelligence is rapidly evolving, and one of the most exciting developments is the emergence of AI agents. As we approach a new era where 2025 is being hailed as the y...
Large language models (LLMs) are undoubtedly powerful—but they come with a catch: a knowledge cutoff. If a fact wasn’t in the training data or if it’s something new (like who won the 2025 Best Pic...